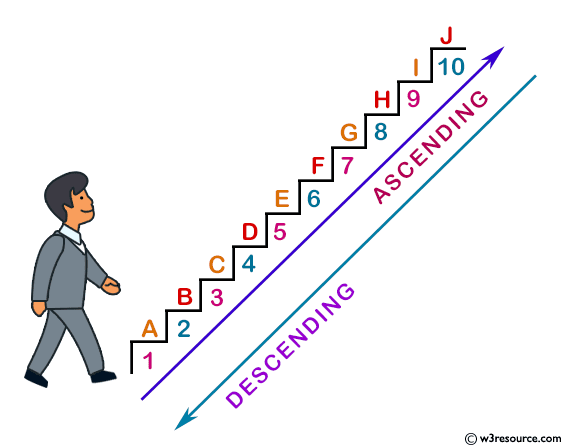
Create an ascending and descending sort function
4 min read
Prequisite:
- Basic understanding of Javascript
- Should at least understand the use of types within Typescript
You will often see on many sites containing some sort of list or table, that it is organised in a particular order, could be by title order, name order or even date order. They will sometimes give you a select button to change the order too. But creating a sort function is not straightforward. We are going to focus on creating an alphabetical sort function.
One of the most important aspects of creating any function, is knowing what data type and data structure is entering the function. And commonly, to have a sort ordered data, the data should ideally be an array of objects literal, so we are going to follow that convention.
Imagine we have an array of objects, like this one below:
[{id: 1,name: "Rechad"},{id: 2,name: "Salma"},{id: 3,name: "Kratos"},{id: 4,name: "Darwin"},]
If we want to order the objects by name alphabetically, we would need to use the sort() method. In our function, we should also make two arguments. One is the array of objects, and the second is the targeted property we want to sort in order, and in our case we will be sorting the name property. Two functions are also required, the ascending function and the descending function.
// Ascending order function (a b c)type Props = {[allOtherProperties: string]: any;};
function ascendingOrder(arrayOfObjects: Array<Props>,targetedProperty: string) {const newArray = [...arrayOfObjects];
newArray.sort((a: Props, b: Props) => {a[targetedProperty].toLowerCase() < b[targetedProperty].toLowerCase() ? -1: 1;});
return newArray};
/∗∗ Expected output:[{id: 4, name: "Darwin"},{id: 3, name: "Kratos"}, {id: 1, name: "Rechad"},{id: 2, name: "Salma"}]∗/
As you can see, it will return the same array of objects, but in ascending order, and you can now display the list with the help of loops. Now the descending function is exactly the same, but switch from less than operator to more than operator.
// Descending order function (c b a)type Props = {[allOtherProperties: string]: any;};
function ascendingOrder(arrayOfObjects: Array<Props>,targetedProperty: string) {const newArray = [...arrayOfObjects];
newArray.sort((a: Props, b: Props) => {a[targetedProperty].toLowerCase() > b[targetedProperty].toLowerCase() ? -1: 1;});
return newArray};
/∗∗ Expected output:[{id: 2, name: "Salma"}{id: 1, name: "Rechad"},{id: 3, name: "Kratos"}, {id: 4, name: "Darwin"},]∗/